Connecting to an Exchange Online PowerShell session is not a complicated task. There are several Microsoft guides that walk you through this, but if you are someone who connects frequently to Exchange Online, the process can be simplified even further with the addition of a PowerShell Custom function to your PowerShell Profile.
Click here to collapse the What is a PowerShell Profile section and skip straight to the instructions for setting up your PowerShell custom functions.
What is a PowerShell Profile?
A PowerShell profile is a PowerShell script that runs automatically when a new PowerShell session is started. PowerShell profiles can be used to configure your PowerShell environment the way you like it, or to load custom functions for use in your PowerShell administration tasks.
You can check to see if you already have a PowerShell profile by opening PowerShell (NOT as admin, as your user) and running the following command:
Test-Path $profile
- If it returns
False
then you do not have a profile. - If a path and file name is returned, then you do have an existing profile.
Creating your PowerShell Profile
If you do not have a PowerShell Profile and would like to create one, you can do so by running the following commands:
New-Item $profile -ItemType File -Force
powershell_ise.exe $profile
- This will open the PowerShell ISE and allow you to paste in the functions you’d like to automatically load when you open PowerShell.
Adding the Connect-EXOnline Function via pre-built script
In order to make this as simple as possible, a PowerShell script is linked below that will automatically check if you already have a Profile and create one if not, then add the necessary functions to your new profile. If you already have a profile but do not have the functions, it will add them for you.
Run the script by extracting the zip file, opening a PowerShell session (Not as Admin) changing directory to the extracted folder containing the AddEXOnlineProfileFunctions.ps1
file, and running the command below:
.\AddEXOnlineProfileFunctions.ps1
- It should display a result message in green text letting you know it was successful.
If you’re curious what that PS1 file contains, here is the raw code:
$profilestatus = Test-Path $PROFILE $functionexists = Get-Content $PROFILE | Select-String "Function Connect-EXOnline" if ($profilestatus -and ($functionexists -notlike "Function Connect-EXOnline")) { Write-Host "You have an existing profile. - Adding the Connect-EXOnline and Disconnect-EXOnline functions now." -ForegroundColor Green Add-Content -Path $PROFILE -Value '' -Force Add-Content -Path $PROFILE -Value 'Function Connect-EXOnline' -Force Add-Content -Path $PROFILE -Value ' {' -Force Add-Content -Path $PROFILE -Value ' $URL = "https://ps.outlook.com/powershell"' -Force Add-Content -Path $PROFILE -Value ' $Credentials = Get-Credential -Message "Enter your Office 365 admin credentials"' -Force Add-Content -Path $PROFILE -Value ' $EXOSession = New-PSSession -ConfigurationName Microsoft.Exchange -ConnectionUri $URL -Credential $Credentials -Authentication Basic -AllowRedirection -Name "Exchange Online"' -Force Add-Content -Path $PROFILE -Value ' Import-PSSession $EXOSession' -Force Add-Content -Path $PROFILE -Value ' }' -Force Add-Content -Path $PROFILE -Value '' -Force Add-Content -Path $PROFILE -Value 'Function Disconnect-EXOnline' -Force Add-Content -Path $PROFILE -Value ' {' -Force Add-Content -Path $PROFILE -Value ' Remove-PSSession -Name "Exchange Online"' -Force Add-Content -Path $PROFILE -Value ' }' -Force Add-Content -Path $PROFILE -Value '' -Force Write-Host "Functions successfully added." -ForegroundColor Green } elseif ($profilestatus -and ($functionexists -like "Function Connect-EXOnline")) { Write-Host "You already have the EXOnline Functions." -ForegroundColor Green } else { Write-Host "You did not have an existing profile file. - Creating your profile file and adding the Connect-EXOnline and Disconnect-EXOnline functions now." -ForegroundColor Green New-Item -Type File -Path $PROFILE -Force Add-Content -Path $PROFILE -Value 'Function Connect-EXOnline' -Force Add-Content -Path $PROFILE -Value ' {' -Force Add-Content -Path $PROFILE -Value ' $URL = "https://ps.outlook.com/powershell"' -Force Add-Content -Path $PROFILE -Value ' $Credentials = Get-Credential -Message "Enter your Office 365 admin credentials"' -Force Add-Content -Path $PROFILE -Value ' $EXOSession = New-PSSession -ConfigurationName Microsoft.Exchange -ConnectionUri $URL -Credential $Credentials -Authentication Basic -AllowRedirection -Name "Exchange Online"' -Force Add-Content -Path $PROFILE -Value ' Import-PSSession $EXOSession' -Force Add-Content -Path $PROFILE -Value ' }' -Force Add-Content -Path $PROFILE -Value '' -Force Add-Content -Path $PROFILE -Value 'Function Disconnect-EXOnline' -Force Add-Content -Path $PROFILE -Value ' {' -Force Add-Content -Path $PROFILE -Value ' Remove-PSSession -Name "Exchange Online"' -Force Add-Content -Path $PROFILE -Value ' }' -Force Add-Content -Path $PROFILE -Value '' -Force Write-Host "Profile file created and functions successfully added." -ForegroundColor Green Write-Host "Here is what your new profile functions look like:" -ForegroundColor Green Write-Host "`n" cat $profile | Write-Host -ForegroundColor Yellow -BackgroundColor Black }
Manually Install the Connect-EXOnline functions
To add the Connect-EXOnline function, we will first open our Profile via the steps above and paste in the following code:
Function Connect-EXOnline { $URL = "https://ps.outlook.com/powershell" $Credentials = Get-Credential -Message "Enter your Office 365 admin credentials" $EXOSession = New-PSSession -ConfigurationName Microsoft.Exchange -ConnectionUri $URL -Credential $Credentials -Authentication Basic -AllowRedirection -Name "Exchange Online" Import-PSSession $EXOSession } Function Disconnect-EXOnline { Remove-PSSession -Name "Exchange Online" }
Save ISE and close out, then open a new PowerShell session and run the following command:
Connect-EXOnline
- A dialog will popup asking for your Office 365 admin credentials. After a short wait the connection will be established and you can run cmdlets such as Get-Mailbox to list the mailbox users in Exchange Online for your Office 365 tenant.
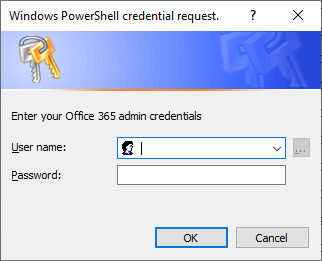
Comments